最近面试了几家美帝大公司的front end职位。在这几次面试中,几乎每次面试的css题目中都有涉及到flexbox的layout。可以看出,flexbox在工业界的使用还是很广泛的。(我几乎没有被问到关于float的问题)
这篇文章会总结flexbox的常用技巧,以及适用场景。未来会添加grid system的信息和内容。
本文的实例参考自css grid vs flexbox
两个例子
用于整个页面的layout
假设我们有如下的page design:
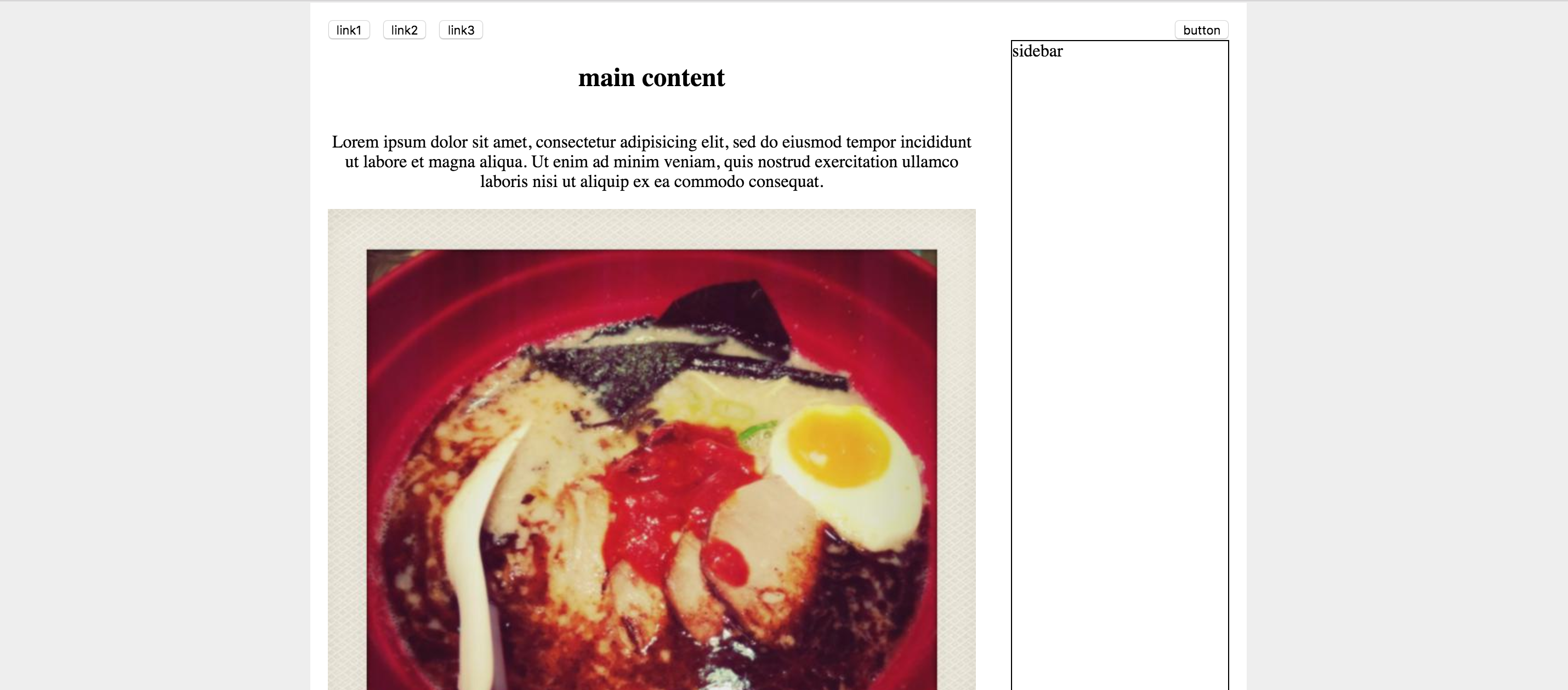
我们可以看到,page上有navbar,main content(分左右两栏),以及footer(未包含在截图中)。这样的layout下可以使用flexbox吗?
当然可以!通过以下几个步骤,即可实现一个简单的layout:
|
|
用于某一个小的component的layout
在上例,我们应用flexbox实现一个页面的整体layout。
如果我们想做card container的layout,且使其支持responsive design,(design如下图,直接用了Thumbtack网站上的card design截图 🙃)
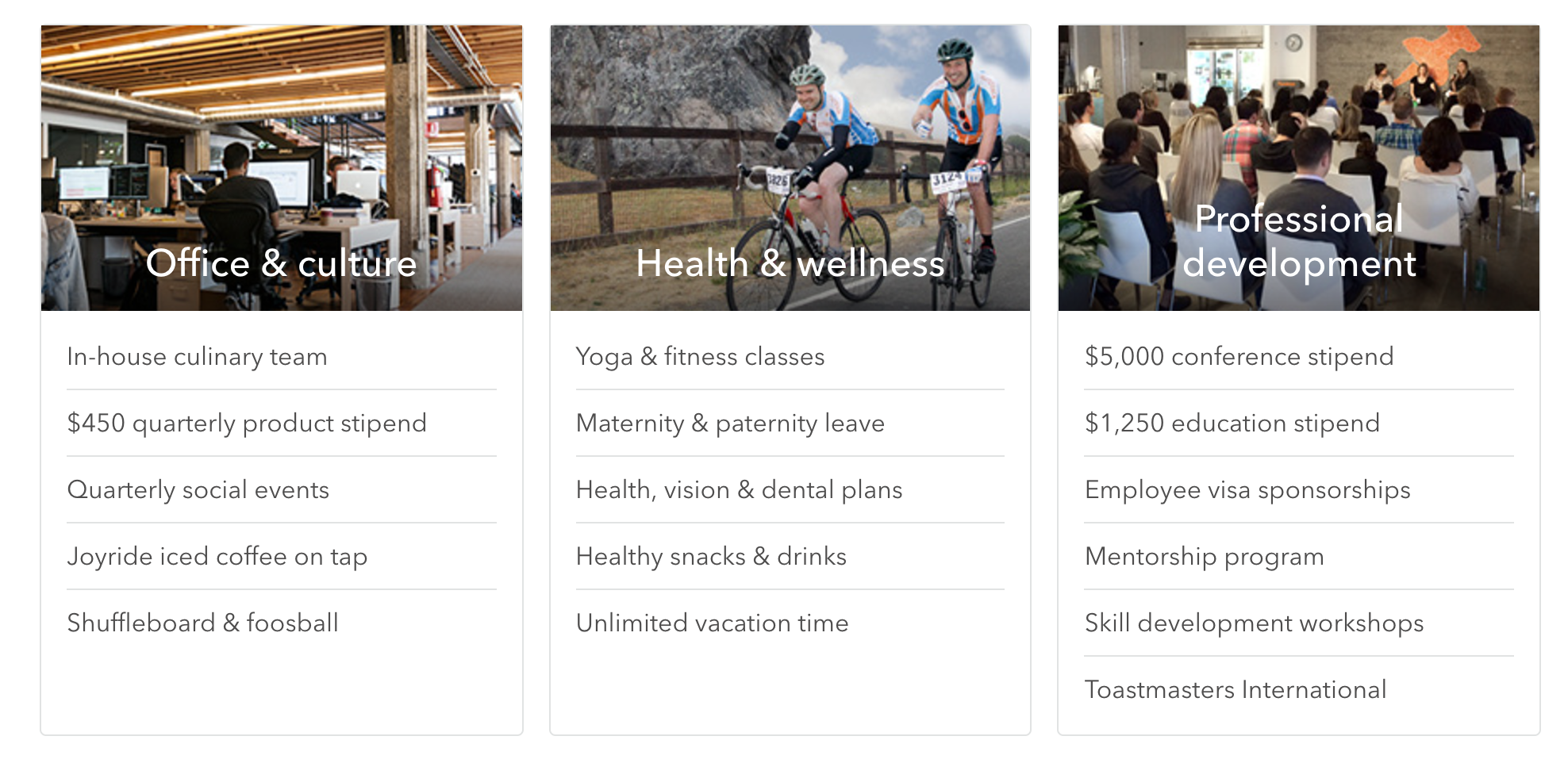
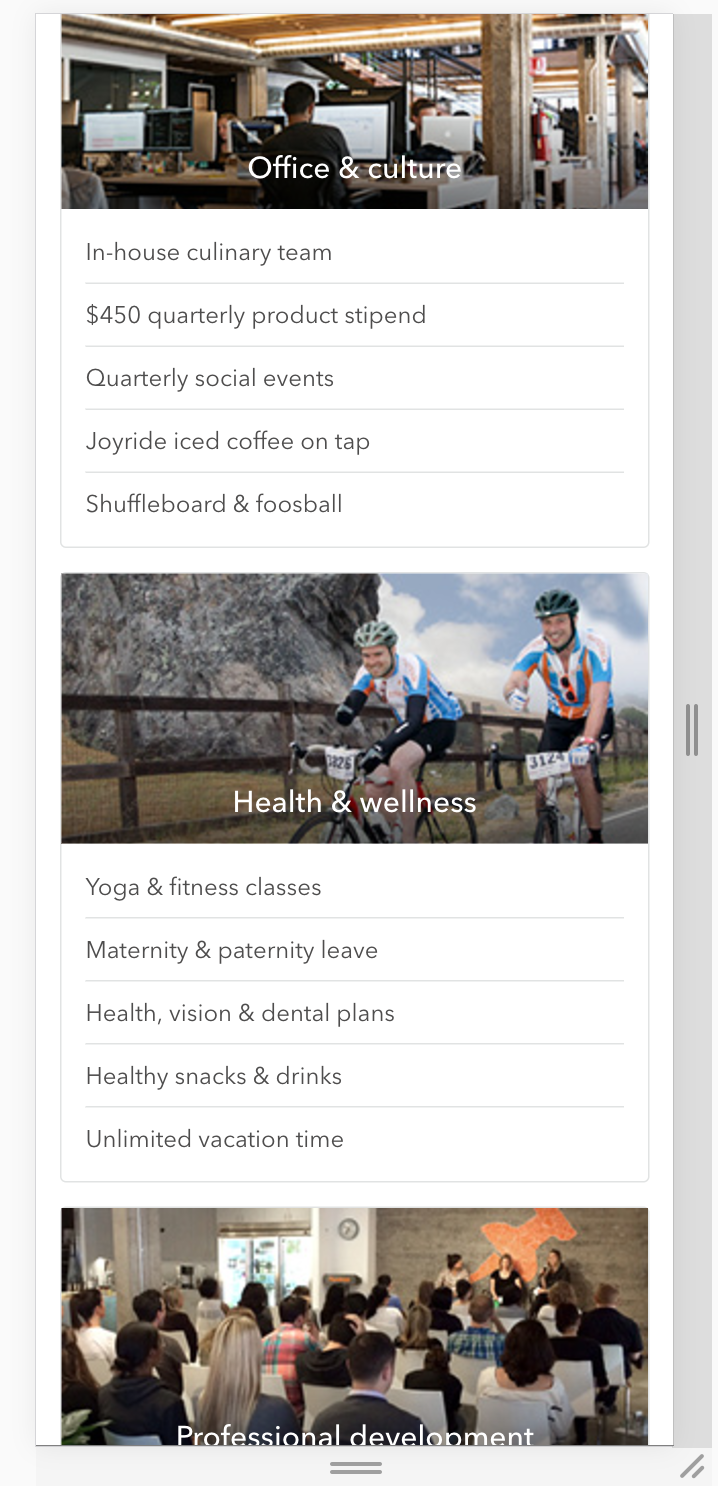
以上layout也可以使用flexbox,且非常简便。
具体的实现如下:
|
|
|
|
在使用flexbox时有几个小技巧:
- 当需要设置一个box内的flex component的宽度比时,灵活使用
flex
。flex
是flex-grow
,flex-shrink
和flex-basis
的简略写法。 align-items
可以纵向对其flex-wrap
可以强制所有element在一行,或是分成几行。同media-query一起灵活使用,可以实现responsive design
Codepen实例如下:
See the Pen ybjXZQ by Zhe Chen (@chenzhe142) on CodePen.
小结
flexbox非常简单易用,且可以实现非常棒的layout效果,对比float,减少了大量的css的使用,也使代码更易维护。唯一的缺点可以说是实现responsive design,运用media-query时还比较繁琐,需要一点一点地精确调整margin,padding或者其他property的值。
综上所述,flexbox非常强大,值得我们深入学习和应用。